If you have a LiveLink™ for Excel® license, then the following methods are available for reading and writing Microsoft Excel Workbook files:
Each row of the spreadsheet represents a different component. The first column can contain a letter, either B or C, denoting that the component can be modeled as either a Block or a
Cylinder primitive. The next column is the total heat dissipation within the component (measured in watts). The next three columns represent the location of the component in the global Cartesian coordinate system (measured in millimeters). Lastly, if the row contains a block component, there are three more columns that denote the width, depth, and height of the block. If the row contains a cylinder component, then there are two more columns that contain the radius and height information, respectively.
The Settings window for the
File Import form object is shown in the figure below.
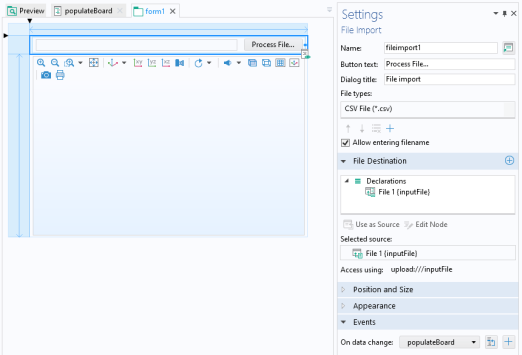
In the Settings window,
CSV File (*.csv) is added to the
File types list. When browsing for the file, this setting will filter out any file that is not a CSV-file.
There is also a File Declaration called
File 1, which is referenced by the file scheme syntax
upload:///inputFile in the method
populateBoard, which is used to read and process the data. The method is called as an event shown at the bottom of the
Settings window of the
File Import form object in the
Events section.
Note that if you would like to open a file browser from a button or a menu item, instead of using a File Import object, you can create a method that calls the built-in method
importFile; for example
The method populateBoard is listed below.
In the first line, the data read from the CSV-file is stored in the 2D array D. The rest of the code parses this array and populates the various parts of a model object. The application allows you to save the result as an MPH-file with variables defined for the heat sources and geometry objects defined for the components, as shown in the figures below.
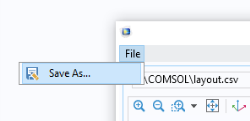
The application described above can easily be extended to also read Microsoft Excel® Workbook files. Note that this requires LiveLink™
for Excel®. In the
Settings window for the
File Import form object, you can add
Microsoft Excel Workbook (*.xlsx) and
Microsoft Excel Workbook (*.xls) to the
File types section, as shown in the figure below.
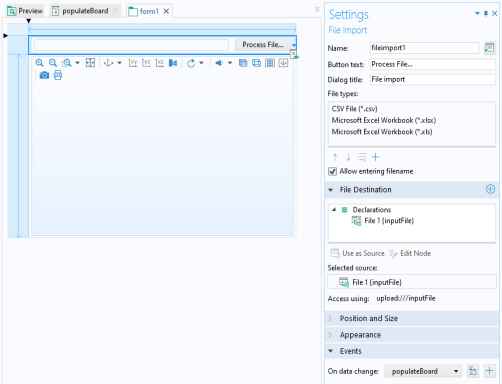
The 2D array D can be defined as a global array in the
Declarations node in the application tree. Alternatively, it can be declared as an array that is local to the method by adding the line
before the if statement. Which option to choose depends on how you would like to use the 2D array data after having read the file.
The method getFilePath returns the full path and name of the uploaded file. The if statements control which method is used to read the file based on its file extension. The file extension is retrieved with the Java® method
endsWith(), which belongs to the
String class. Note that you can see which methods are available for a string by typing the name of the string followed by a period and Ctrl+Space, as shown in the figure below.
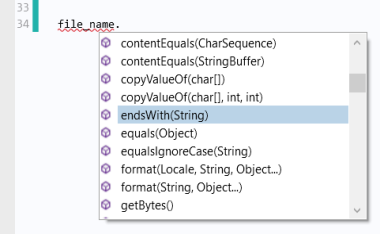
You can write to a CSV-file using four different call syntaxes for the method writeCSVFile, depending on if the contents are strings or doubles and whether you would like to overwrite an already existing file or appending to its contents.
This corresponds to a change in the y-coordinate of one of the blocks from
150 to
140.
The first line stores the size of the 2D array (or matrix) D in a 1-by-2 array (or vector) sz. The second line sets the string value of the
y-coordinate of the block of the second-to-last row in
D.
writes the data to a file my_layout.csv in a temporary folder whose location is determined by the Preferences of either COMSOL Multiphysics or COMSOL Server, depending on which software is used to run the application. For example, in a typical Windows® installation of COMSOL Multiphysics, the location will be similar to
Note that as a first step in the example above, the file is written to a temporary file using the writeCSVFile method. This step is done automatically by the application. In the second step, the method
fileSaveAs opens a file browser and lets the user of the application choose the file location; for example, a folder on the computer’s local file system or to a network folder. This extra step is needed in order for the application to function in a web browser. Due to the security settings of a typical web browser, the application is not permitted to automatically save a file to an arbitrary location. Instead, the application is allowed to save to a few specific locations, including the
temp folder, whose location is specified in the
Preferences settings. The other locations are the
user and
common folders, also specified in the
Preferences settings. For more information, see the
Introduction to Application Builder.
If you have licensed LiveLink™ for Excel®, then you can write to a Microsoft Excel Workbook file in a way that is similar to that of a CSV-file, with the exception that the append option is not available. The following code, corresponding to the previous CSV-file example, shows how to write to an Excel file.
Reading files with numerical data in matrix format is easiest when using the readMatrixFromFile method. This method assumes that the file has the spreadsheet format, as available in the model tree
Export node. The example below shows a file on the spreadsheet format.
The first few lines with comments start with the character % and are ignored by the
readMatrixFromFile method. You can optionally omit such lines and just have the numerical part of a file read by
readMatrixFromFile. Assume that this file is uploaded to an application using a
File Import form object and a
File declaration
file1. The following code can then be used to read the data into a double array
p.
Reading files on the spreadsheet format as a string array can be done with the method readStringMatrixFromFile. Also, in this case, the comment lines will be ignored. The code below shows how you can replace the first few lines in the above example using
readStringMatrixFromFile instead of
readMatrixFromFile.
The method readStringMatrixFromFile is most useful when parts of the read file contains text.
To write numerical matrix data to file, you can use the method writeFile. Assume that you want to write a matrix of random 2D coordinate values to a file on the spreadsheet format; for example:
If you would like to export a matrix with a mix of numeric and text data, you can use the writeFile method with a string array instead of a double array. The syntax for this case is otherwise identical to that of the double array shown in the example above.
For reading text files into a string, you can use the method readFile. A straightforward use of
readFile is for previewing a text file; for example, before importing and parsing it, as illustrated by the example application in the figure below.
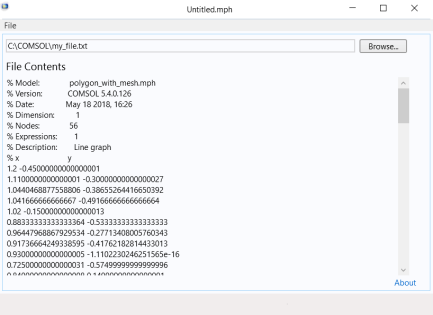
This application has two form objects: a File Import form object referencing a
File declaration
file1 and a
Text form object referencing a string
str declared in the
Declarations node as a global variable.
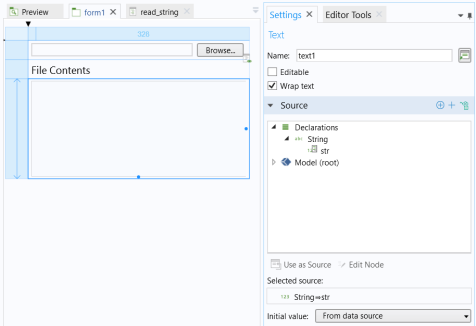
The File Import form object has an
Event that calls the method
read_string upon data change.
Since the Text object is referencing the global string
str, the contents of the file are displayed in the
Text object immediately after import.
Parsing of smaller text files can be done with readFile in combination with the many text processing methods available in the
String class. However, it is often more efficient to use methods in the
CsReader class, as described in the section
“Processing Text Files using the CsReader and CsWriter Classes” on page 182, especially for larger text files. The reason is that when using the
readFile method, the entire file is read into a string with all its contents kept in memory; whereas when using the
CsReader class methods, only small portions of the file are kept in memory at any given time.
If you would like to parse smaller text files using readFile, then the built-in
“String Methods” on page 150 are useful. The example code below illustrates using the built-in methods
findIn,
substring,
split, as well as the regular Java® methods
System.getProperty and
String.startsWith. The example parses the header of a text file containing polygon information to retrieve information about the number of points of each polygon in the main body of the file (not shown) as well as the number of properties (for example, color or material property). The header portion of the file may look like the example below.
The code for parsing the header is listed below. It stores the number of points and properties in the variables n_of_points and
n_of_properties, respectively. To keep things simple, no error handling is done. For example, the code assumes that there is exactly one instance of
begin_header and
end_header.
The Java® String class has many methods for text processing. See the online Java® documentation for more information.
The use of the file scheme syntax temp:/// is described above in the earlier examples of this section. The end-of-line characters of this example are for Windows®; see also
“Special Characters” on page 11.
The most efficient and flexible way to read and write to a text file is to use the methods in the CsReader and
CsWriter classes, respectively. However, using the methods of these classes is more complicated than using any of the built-in methods described above.
The CsReader class inherits all public methods of the abstract Java® class
Reader. In a similar way, the
CsWriter class inherits all public methods of the abstract Java® class
Writer. This means that when using these classes, you get access to a large number of methods for processing text files. These methods are not documented here, but you can find a lot of information with regards to using these methods online as well as in books on Java® programming. In addition, you can see which methods are available by using code-completion Ctrl+Space.
which is reading a line from the character stream and storing the result in the string line. A line is considered to be terminated by one of the characters carriage return
\r, line feed
\n, or the composite
\r\n. If there are no more lines to read, then
null is returned.
The example of the section “Reading Matrix Files” on page 177 uses an example on the spreadsheet data format. This section contains an example that writes a file on the sectionwise format, the other primary format in COMSOL Multiphysics for saving postprocessing data. Data on the sectionwise format can, for example, be read into an
Interpolation Curve geometry primitive. A file on the sectionwise format may look like this:
The first few lines with comments start with the character % and are ignored when imported as an
Interpolation Curve. The first section containing data starts on the line after
% Coordinates. The second section containing data starts on the line after
% Elements (segments). Note that the strings
Coordinates and
Elements (segments) are not necessary but each section containing data will be assumed to start after each block of comments, regardless of what comes after the character
%. There may be additional blocks of data when, for example, exporting
Contour plot data.
The following example code uses a CsWriter stream to write interpolation curve data to a text file. A template point set
p is copied in a circular pattern for a given radius
R and number of copies
n_of_copies.
appends the contents of the string header to the (empty) file
my_curve.txt.
Just as described above for the reader object, you can see which additional methods are available for the
writer object by using Ctrl+Space, as shown in the figure below.
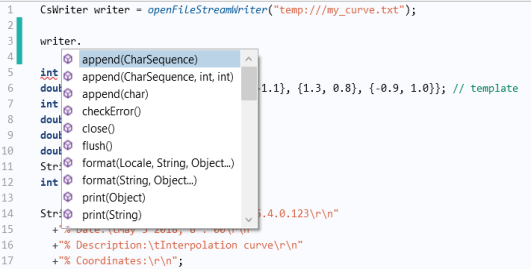
You can import the resulting interpolation data as an Interpolation Curve by selecting the
Sectionwise option for
Data format. This can be done for a 2D geometry object or for a
Work Plane in 3D. The figure below shows the data imported to a 2D model.
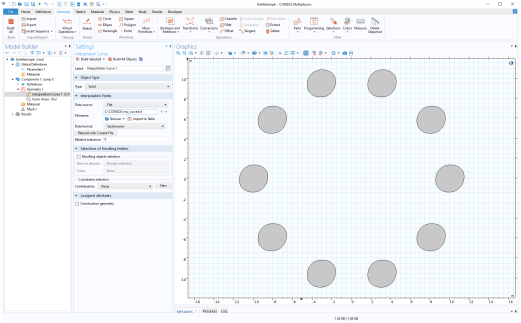
You write data to a binary file by using the methods of the class CsBinaryWriter in a way that is somewhat similar to that of writing text using
CsWriter. However, instead of writing strings and characters, you are writing bytes. To see how many bytes each data type requires, see the table in the section
“Primitive Data Types” on page 8.
The example code below writes random 3D point data to a binary file. Each point coordinate is stored as a double and takes 8 bytes to store. The first 4 bytes of the file stores the number of points in the file as an
int.
To conveniently convert between the regular data types, such as double,
int, and
byte arrays, the Java® library method
java.nio.ByteBuffer is needed. This method is not part of the standard methods available in the Method Editor and you need to use the fully qualified Java® class name
java.nio.ByteBuffer, as shown in the example code below.
uses the imported ByteBuffer method to convert the integer
N to a byte array
bytes4 of length 4.
The for-loop creates
N points and writes each
x-,
y-, and
z-coordinate as doubles using a byte array
bytes8 of length 8.
opens a Java® byte stream based on a File declaration
file1, typically referenced in a
File Browser form object, as in the earlier examples on reading text files.
The following for-loop reads chunks of 8 bytes into the byte array
bytes8, converts them and stores the results in a 2D double array
p.