The Size attribute provides a number of input properties that can control the mesh element size, such as the following properties:
To override this behavior, set hauto to another integer. Override this by setting specific size properties, for example, making the mesh finer than the default by specifying a maximum element size of 0.02:
To create a structured quadrilateral mesh in 2D, use the Map operation. This operation uses a mapping technique to create the quadrilateral mesh.
Use the EdgeGroup attribute to group the edges (boundaries) into four edge groups, one for each edge of the logical mesh. To control the edge element distribution use the
Distribution attribute, which determines the overall mesh density.
The EdgeGroup attributes specify that the four edges enclosing domain 1 are boundaries 1 and 3; boundary 2; boundary 8; and boundary 4. For domain 2 the four edges are boundary 4; boundary 5; boundary 7; and boundaries 9, 10, and 6.
The final mesh is in Figure 3-7. Note the effect of the
Distribution feature, with which the distribution of vertex elements along geometry edges can be controlled.
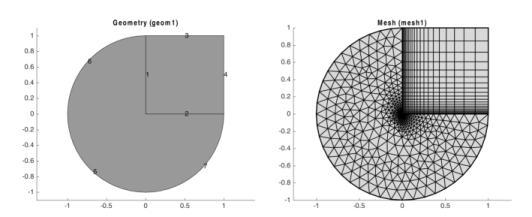
Thus, to get the FreeQuad feature before the
FreeTri feature, the
dis1 feature needs to be made the current feature by building it with the
run method. Alternatively, parts of a mesh can be selectively removed by using the
Delete feature. For example, to remove the structured mesh from domain 2 (along with the adjacent edge mesh on edges 3 and 4), and replace it with an unstructured quad mesh, enter these commands:
Create 3D volume meshes by extruding and revolving face meshes with the Sweep feature. Depending on the 2D mesh type, the 3D meshes can be hexahedral (brick) meshes or prism meshes.
To obtain a torus, leave the angles property unspecified; the default value gives a complete revolution.
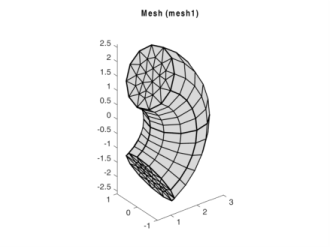
The result is shown in Figure 3-9. With the properties
elemcount and
elemratio the number and distribution of mesh element layers is controlled in the extruded direction.
For 2D and 3D geometries it is also possible to create boundary layer meshes using the BndLayer feature. A boundary layer mesh is a mesh with dense element distribution in the normal direction along specific boundaries. This type of mesh is typically used for fluid flow problems to resolve the thin boundary layers along the no-slip boundaries. In 2D, a layered quadrilateral mesh is used along the specified no-slip boundaries. In 3D, a layered prism mesh or hexahedral mesh is used depending on whether the corresponding boundary layer boundaries contain a triangular or a quadrilateral mesh.
Given a mesh consisting only of simplex elements (lines, triangles, and tetrahedra) you can create a finer mesh using the feature
Refine. Enter this command to refine the mesh:
By specifying the property tri, either as a row vector of element numbers or a 2-row matrix, the elements to be refined can be controlled. In the latter case, the second row of the matrix specifies the number of refinements for the corresponding element.
The refinement method is controlled by the property rmethod. In 2D, its default value is
regular, corresponding to regular refinement, in which each specified triangular element is divided into four triangles of the same shape. Setting
rmethod to
longest gives longest edge refinement, where the longest edge of a triangle is bisected. Some triangles outside the specified set might also be refined in order to preserve the triangulation and its quality.
In 3D, the default refinement method is longest, while regular refinement is only implemented for uniform refinements. In 1D, the function always uses
regular refinement, where each element is divided into two elements of the same shape.
Use the CopyEdge feature in 2D and the
CopyFace feature in 3D to copy a mesh between boundaries.
Use the Convert feature to convert meshes containing quadrilateral, hexahedral, or prism elements into triangular meshes and tetrahedral meshes. In 2D, the function splits each quadrilateral element into either two or four triangles. In 3D, it converts each prism into three tetrahedral elements and each hexahedral element into five, six, or 28 tetrahedral elements. To control the method used to convert the elements, use the property
splitmethod.